In this project I’ll show you how to compare directories, save the result, and process the result: all combined in one attractive GUI.
The first thing we’ll do: we have to find the right tools/methods in Python:
so I’ve googled on “python compare directories” and found this one on the official documentation:
https://docs.python.org/3/library/filecmp.html
Now let’s start! First we’ll create the project:
Create “CompareDirectories” Project
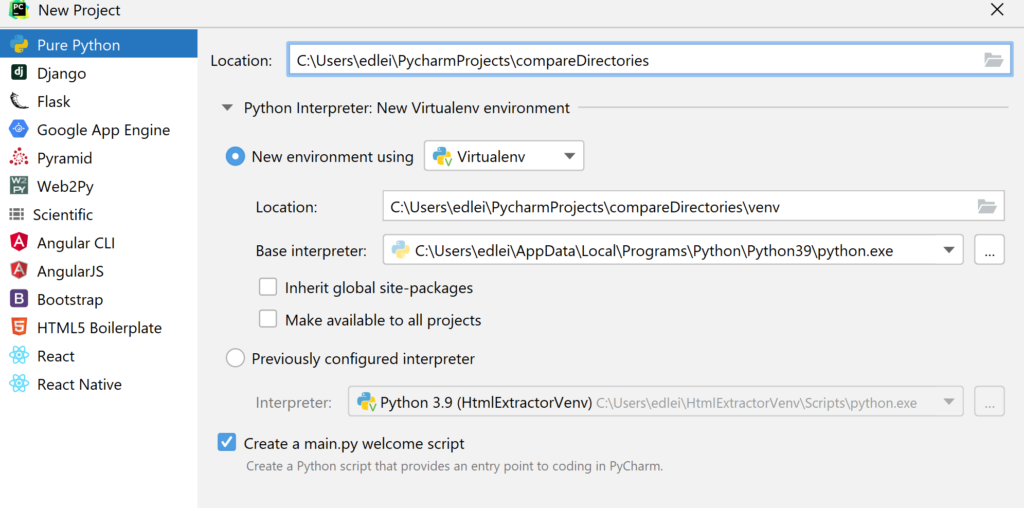
Share project on Github (optional, recommended)
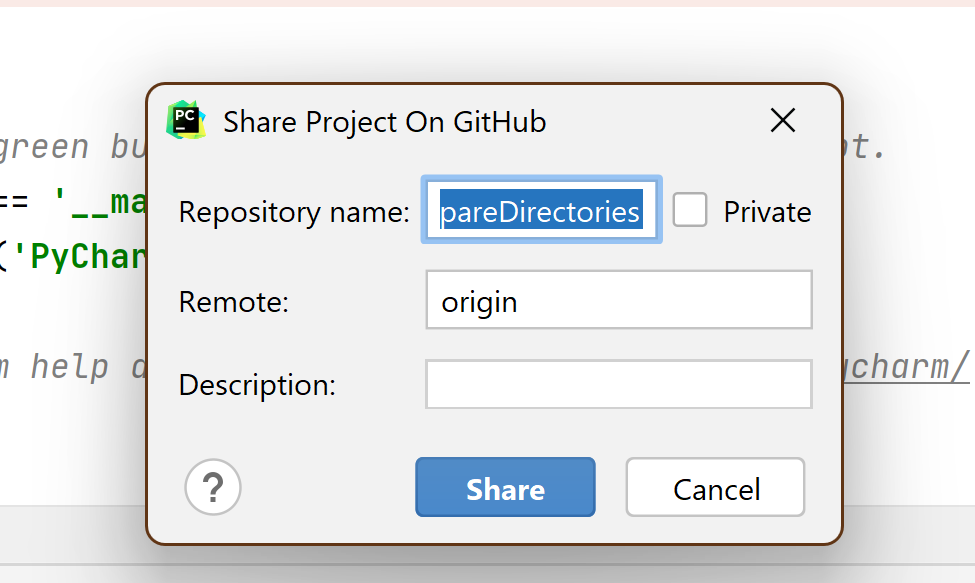
Initial commit
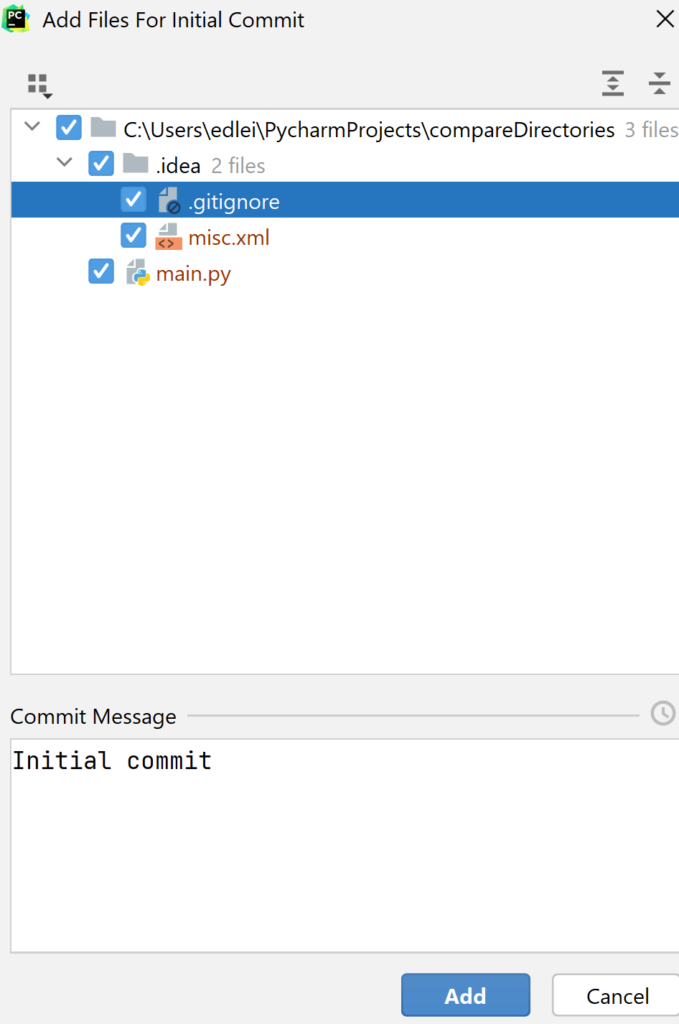
Now we have an initial, almost empty “compareDirectories” project on Github. It will serve us as a backup as well.
We will open the project directly on Github now and we’ll add a readme to it:
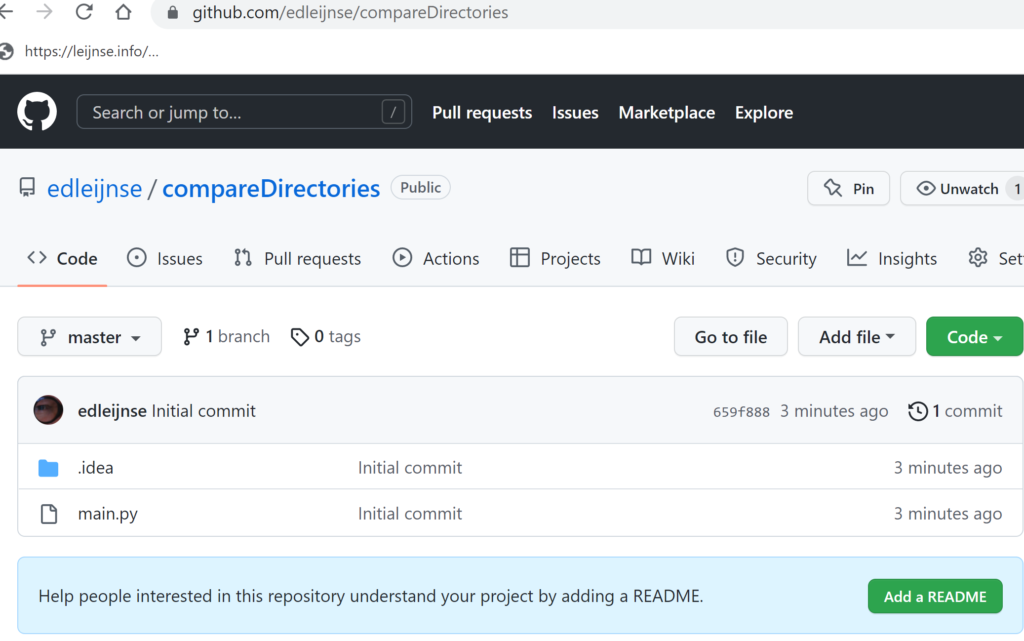

Now we’re done and we can update our project in Pycharm (with pull).
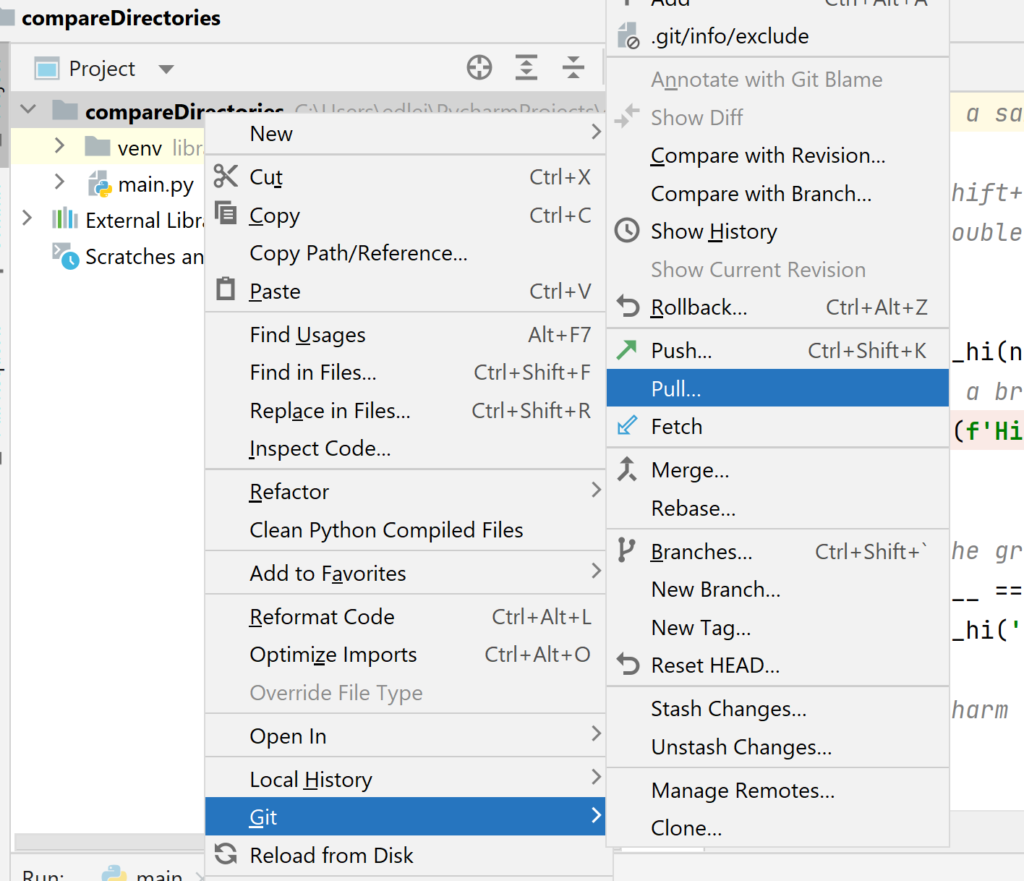
Now it’s time to really start!
We will start with a “first try” copied from
https://docs.python.org/3/library/filecmp.html
from filecmp import dircmp def print_diff_files(dcmp): for name in dcmp.diff_files: print("diff_file %s found in %s and %s" % (name, dcmp.left, dcmp.right)) for sub_dcmp in dcmp.subdirs.values(): print_diff_files(sub_dcmp) dcmp = dircmp('dir1', 'dir2') print_diff_files(dcmp)
I’ve changed the code like this:
def print_diff_files(dcmp):
common_files = sorted(dcmp.common_files)
for name in common_files:
print("common_file %s found in %s and %s" % (name, dcmp.left,
dcmp.right))
left_only_files = sorted(dcmp.left_only)
for name in left_only_files:
print("left_only_file %s found in %s" % (name, dcmp.left))
right_only_files = sorted(dcmp.right_only)
for name in right_only_files:
print("right_only_file %s found in %s " % (name, dcmp.left))
for sub_dcmp in dcmp.subdirs.values():
print_diff_files(sub_dcmp)
What is really amazing to me is the sheer amount of information you’ll get from this compare method: try to experiment with it in debug mode and you’ll see what I mean.
Start with the GUI first
We’ll build a very simple GUI to choose both directories. Afterward we’ll add an action to it.
As a base project we will use this one:
https://github.com/edleijnse/pythonGui
You’ll find the gui part in TaggerBizControlCentre.py
Now I’ve made a first button “compare from”:
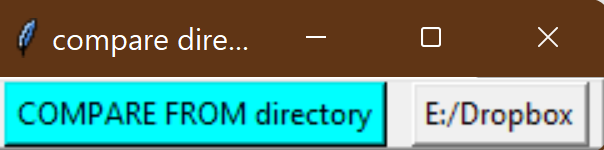
self.btn_compare_from_dir = tk.Button(self.fr_buttons, text="COMPARE FROM directory",
command=compareFromClicked, highlightbackground="cyan", bg="cyan")
While defining the button you can add a command to it, in this case: “compareFromClicked”.
In the command you can define what happens after you clicked the button.
def compareFromClicked():
print("compare from clicked")
mydir = open_directory(self.filearray[0], "directory to compare from")
btn_compare_from_dir_txt_var.set(mydir)
self.filearray[0]=mydir
The programmed action is like this: the program will ask you where to find the directory and store the value in 2 variabels: the first “btn_compare_from_dir_txt_var” is displayed as a label field right from the button. the second “self.filearray” is used to save the chosen directory.
In the same style I’ve added a “compare_to” button.
And I’ve added 2 methods for saving and reading the data in a file called
compareDirectoriesconfig.txt
So, now we have some basics to start.